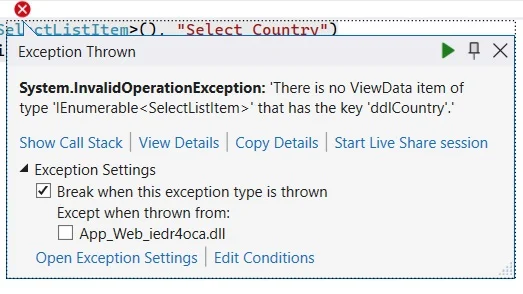
In this article, we try to find out why we are getting this error, i.e., "there is no viewdata item of type IEnumerable <selectlistitem> that has the key," whenever we bind the data into a drop-down list, and also figure out how to resolve this issue.
First of all, I want to make it clear, that whenever we try to bind data from the backend to the dropdown list, that dropdown list will expect an enumerable list of SelectListItems to bind.
Error Reasons:
ViewBag.CountryList = null;
or
ViewData["CountryList"]=null;
@Html.DropDownList("ddlCountry2", ViewBag.CountriesList as IEnumerable<SelectListItem>, "Select Country")
or
@Html.DropDownList("ddlCountry2", ViewData["CountryLists"] as IEnumerable<SelectListItem>, "Select Country")
Add Controller: CountryController.cs
Adding controller with the name of CountryController class, and add necessary action methods.
using System.Web.Mvc;
public class CountryController : Controller
{
// ActionMethod code
}
adding action method with the name of Index, you can see below the code
public ActionResult Index()
{
return View();
}
Adding Dropdownlist in Index.cshtml
After creating the Index Action Method simultaneously, I added the Index.cshtml view page. In the index.cshtml view page, I added a drop-down list without binding any data.
@Html.DropDownList("ddlCountry", "Select Country")
Now, if you run the application, you get the same error as usual. Because that dropdown list is expecting the IEnumerable SelectListItem object, and we are not passing any object, even though we are not telling the dropdown list that we don't have any data to bind in the dropdown list control.
Binding Empty Dropdownlist
When the dropdown list is expecting data from us, but we don't have any data to bind. In that scenario, we can tell the dropdown list that we have an empty selectlistitem list object.
@Html.DropDownList("ddlCountry",Enumerable.Empty<SelectListItem>(),"Select Country")
Enumerable.Empty<SelectListItem>() its create empty object
Binding ViewBag.CountryList or ViewData["CountryList"] into Dropdownlist
In this requirement, if we want to bind viewbag.countrylist or viewdata["countrylist"] data into the dropdown list on the view page, First, we need to create a List<SelectListItem> object and then assign it to viewbag.countrylist and viewdata["countrylist"].
public ActionResult Index()
{
ViewBag.CountryList = new List<SelectListItem>()
{
new SelectListItem(){Text="India", Value="1"},
new SelectListItem(){Text="France", Value="2"},
new SelectListItem(){Text="Russia", Value="3"},
new SelectListItem(){Text="Japan", Value="4"},
new SelectListItem(){Text="Saudi", Value="5"},
};
ViewData["CountryList"] = new List<SelectListItem>()
{
new SelectListItem(){Text="India", Value="1"},
new SelectListItem(){Text="France", Value="2"},
new SelectListItem(){Text="Russia", Value="3"},
new SelectListItem(){Text="Japan", Value="4"},
new SelectListItem(){Text="Saudi", Value="5"},
};
return View();
}
Each SelectListItem contains text and a value. The text represents the country name, and the value represents the unique ID that we have given for each country.
Let's see how to implement it in the index.cshtml view page.
@Html.DropDownList("ddlCountry2", ViewBag.CountryList as IEnumerable<SelectListItem>, "Select Country")
If you see this code dropdown list in the view page ViewBag.CountryList as IEnumerable<SelectListItem> its type casting to IEnumerable<SelectListItem>, Simillar to ViewData["CountryList"] because both ViewBag and ViewData: viewbag returns dynamic type objects and viewdata returns object types.
Conclusion:
The article aims to make you understand as a fresher what causes this error what the possibilities for getting the same error what the reason is and how to resolve that error.
0 Comments